Introduction
Welcome to the home of Email Hippo technical documents for our ASSESS API. From here you can access full information about the API, view code in multiple languages and get started with your own integration.
About Email Hippo
Email Hippo is an email intelligence and data services provider that’s trusted by thousands of businesses and holds international security accreditations.
We deliver accurate cloud-based email validation and domain profiling. API use cases include data cleansing on demand or in real-time, fraud prevention and user signposting scenarios to improve user experience and build effective communication processes.
Our monitoring and detection of disposable email addresses is unrivalled.
About our APIs
> 99.99% uptime
Fully load balanced and automatic fail-over systems dispersed across multiple data centers in multiple regions deliver enterprise-grade resilience.
Easy integration
Take a look at our enpoints and code samples to see how quick and easy it is to integrate with our services.
Fast, transparent response times
Every query response includes stopwatch data that shows the time taken to execute the request. Typical queries are answered in under 0.5 seconds.
Unrivalled performance
Strategic data centers in Europe, aggressive caching, global network delivery optimization and cloud-based auto-scaling deliver outstanding performance.
Thoughtful versioning
Endpoints are ‘versioned’ allowing the release of new functionality without forcing customers to change or break if they are committed to integrating with legacy endpoints.
Service Status 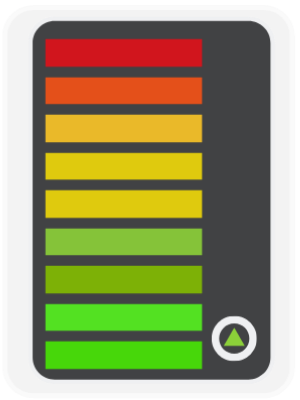
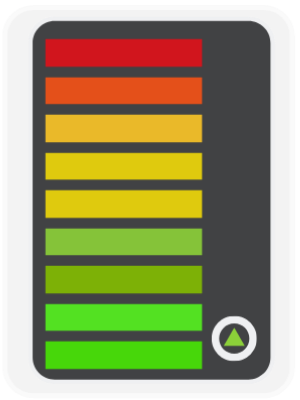
For information on the status and performance of our services visit status.emailhippo.com.
ASSESS Pre-fraud API 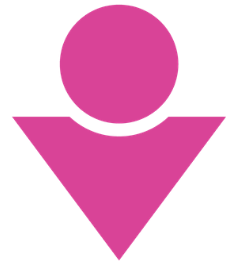
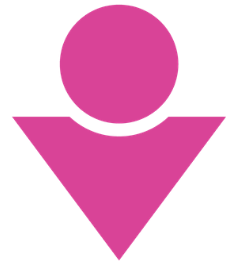
The next generation of the award-winning Email Hippo Trust Score; ASSESS is a scalable pre-fraud system for businesses from entry to enterprise levels. It delivers results via an API that’s integrated at the point where users sign-up and enter an email address. ASSESS explores the data behind every sign-up, looking for ‘tells’ and assigning a numeric level of risk to every result. The aggregated results are produced as a single Trust Score, enabling businesses to detect potentially risky sign-ups and take action accordingly.
Common features:
- Progressive verification using multiple verification processes including:
- Syntax checking
- DNS checking
- Block-list checking (e.g. spamhaus)
- Web infrastructure checking
- Mailbox checking
Unrivalled coverage - Email Hippo leverages the advantages of its scalable infrastructure to provide coverage of domains that are technically challenging. Consumer facing domains tend to be more challenging to cover then business facing domains B2C domains.
Disposable email address detection - Unrivalled disposable email address detection built on Email Hippo’s proprietary multi-vector real-time analysis:
- Real-time detection of disposable email address providers that employ obfuscation techniques (e.g. rotating domains, IP addresses and MX servers)
- Checking against static lists
Gibberish detection
- A common vector for persons wishing to remain anonymous is to register or use a pre-existing domain. Finding an available domain is not easy and as such, many opt for a ‘Gibberish’ domain such as
sdfre45321qaxc.com
. - Email Hippo detects gibberish in both the user and domain elements of an email address.
- A common vector for persons wishing to remain anonymous is to register or use a pre-existing domain. Finding an available domain is not easy and as such, many opt for a ‘Gibberish’ domain such as
Domain age - Email Hippo's WHOIS service contributes valuable domain age information to help evaluate the credibility of domains.
IP address - Email Hippo digs into the IP address to understand its origin and reputation.
Proprietary scoring and risk assessment - All the information is wrapped into our Hippo Trust Score. A simple score between 0 and 10 rates your visitor so you can decide whether and how you treat your visitor.
Consumer or Business assessment - Email Hippo assesses whether the email address is likely to be from a consumer or a business and presents the result as a score from 0 to 10. The closer the score is to 0 the more likely the email address belongs to a consumer, the closer it is to 10 the more likely it is to belong to a business.
ASSESS Edition 1
Specification
Item | Spec |
---|---|
Manufacturer | emailhippo.com |
Current version | v1 |
Uptime | > 99.99% |
Response time | >0.2 seconds < 8 seconds. Typical response time 0.4 seconds. |
Throughput and concurrency | 220 TPS(Transactions Per Second) |
Security and encryption | Transport security using HTTPS. Data at rest encrypted using 256-bit AES encryption. |
Integration | RESTful GET over HTTPS, XML GET over HTTPS, BSON over HTTPS, protobuf over HTTPS |
Authentication | License key |
Infrastructure | Geographically dispersed cloud data centers, auto load balance / failover |
Concurrency
To preserve the operational integrity of the service to all of our customers, there is a maximum concurrency enforced by our systems.
Limits
Allowed throughput is 220 ASSESS requests per second.
Throughput exceeding these limits will receive HTTP response code 429 (too many requests) for subsequent requests for a duration of one minute.
Suggestions on how to manage throughput
If you experience or anticipate exceeding throughput limits, here are two ways to control query rates:
Test your integration with representative production loads over a period of time. Monitor response codes for any 429’s. If you see any 429’s please reduce the rate at which your application is querying our servers.
For applications that can tolerate slight delays in your data processing mesh, consider using queuing infrastructure with a rate controllable processor. Your ‘processor’ can then schedule picking work off the queue and submitting requests to our systems at a controllable rate.
Large throughput requirement
For sustained throughput of more than 220 requests per second, please contact us about a private, dedicated service.
Authentication
Email Hippo ASSESS uses API keys to allow access to the API. API keys can be managed within Hippo World.
Email Hippo ASSESS expects the API key to be included in all API requests to the server.
{k}: yourlicensekey
Endpoint - GET quota usage
* © 2020, Email Hippo Limited. (http://emailhippo.com)
# You can also use wget
curl -X GET https://api.hippoassess.com/v1/quota/{apiKey} \
-H 'Accept: application/json'
/*
* © 2020, Email Hippo Limited. (http://emailhippo.com)
*/
<?php
require 'vendor/autoload.php';
$headers = array(
'Accept' => 'application/json',
);
$client = new \GuzzleHttp\Client();
// Define array of request body
$request_body = array();
try {
$response = $client->request('GET','https://api.hippoassess.com/v1/quota/{apiKey}', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
?>
const headers = {
'Accept':'application/json'
};
fetch('https://api.hippoassess.com/v1/quota/{apiKey}',
{
method: 'GET',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
URL obj = new URL("https://api.hippoassess.com/v1/quota/{apiKey}");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("GET");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
require 'rest-client'
require 'json'
headers = {
'Accept' => 'application/json'
}
result = RestClient.get 'https://api.hippoassess.com/v1/quota/{apiKey}',
params: {
}, headers: headers
p JSON.parse(result)
# © 2020, Email Hippo Limited. (http://emailhippo.com)
import requests
headers = {
'Accept': 'application/json'
}
r = requests.get('https://api.hippoassess.com/v1/quota/{apiKey}', headers = headers)
print(r.json())
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Accept": []string{"application/json"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("GET", "https://api.hippoassess.com/v1/quota/{apiKey}", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
/*
* © 2020, Email Hippo Limited. (http://emailhippo.com)
*/
using System;
using System.Collections.Generic;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Text;
using System.Threading.Tasks;
using Newtonsoft.Json;
/// <<summary>>
/// Example of Http Client
/// <</summary>>
public class HttpExample
{
private HttpClient Client { get; set; }
/// <<summary>>
/// Setup http client
/// <</summary>>
public HttpExample()
{
Client = new HttpClient();
}
/// Make a dummy request
public async Task MakeGetRequest()
{
string url = "https://api.hippoassess.com/v1/quota/{apiKey}";
var result = await GetAsync(url);
}
/// Performs a GET Request
public async Task GetAsync(string url)
{
//Start the request
HttpResponseMessage response = await Client.GetAsync(url);
//Validate result
response.EnsureSuccessStatusCode();
}
/// Deserialize object from request response
private async Task DeserializeObject(HttpResponseMessage response)
{
//Read body
string responseBody = await response.Content.ReadAsStringAsync();
//Deserialize Body to object
var result = JsonConvert.DeserializeObject(responseBody);
}
}
Retrieves the current usage details for a given license key.
GET //api.hippoassess.com/quota/{apiKey}
GET QUOTA record.
Parameters
Parameter | In | Type | Required | Description |
---|---|---|---|---|
apiKey | path | string | true | the license key |
Responses (including errors)
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | Success | QuotaRecord |
400 | Bad Request | Must enter a valid license key to query or invalid format. | None |
404 | Not Found | No data found. | None |
422 | Unprocessable Entity | Cannot process a fully parsed respone. Top Level Domain (TLD) is not supported. | None |
500 | Internal Server Error | Server error. | None |
QuotaRecord schema
{
"quotaUsed": 0,
"quotaRemaining": 0,
"nextQuotaResetDate": "2018-08-09T10:26:42Z",
"reportedDate": "2018-08-09T10:26:42Z"
}
Name | Type | Required | Restrictions | Description |
---|---|---|---|---|
quotaUsed | integer(int64) | false | none | none |
quotaRemaining | integer(int64) | false | none | none |
nextQuotaResetDate | datetime | false | none | none |
reportedDate | datetime | false | none | none |
Endpoint - GET ASSESS result
* © 2020, Email Hippo Limited. (http://emailhippo.com)
# You can also use wget
curl -X GET https://api.hippoassess.com/v1/json/{apiKey}/{emailAddress}/{ipAddress}/{firstName}/{lastName} \
-H 'Accept: application/json'
/*
* © 2020, Email Hippo Limited. (http://emailhippo.com)
*/
<?php
require 'vendor/autoload.php';
$headers = array(
'Accept' => 'application/json',
);
$client = new \GuzzleHttp\Client();
// Define array of request body.
$request_body = array();
try {
$response = $client->request('GET','https://api.hippoassess.com/v1/json/{apiKey}/{emailAddress}/{ipAddress}/{firstName}/{lastName}', array(
'headers' => $headers,
'json' => $request_body,
)
);
print_r($response->getBody()->getContents());
}
catch (\GuzzleHttp\Exception\BadResponseException $e) {
// handle exception or api errors.
print_r($e->getMessage());
}
?>
const headers = {
'Accept':'application/json'
};
fetch('https://api.hippoassess.com/v1/json/{apiKey}/{emailAddress}/{ipAddress}/{firstName}/{lastName}',
{
method: 'GET',
headers: headers
})
.then(function(res) {
return res.json();
}).then(function(body) {
console.log(body);
});
URL obj = new URL("https://api.hippoassess.com/v1/json/{apiKey}/{emailAddress}/{ipAddress}/{firstName}/{lastName}");
HttpURLConnection con = (HttpURLConnection) obj.openConnection();
con.setRequestMethod("GET");
int responseCode = con.getResponseCode();
BufferedReader in = new BufferedReader(
new InputStreamReader(con.getInputStream()));
String inputLine;
StringBuffer response = new StringBuffer();
while ((inputLine = in.readLine()) != null) {
response.append(inputLine);
}
in.close();
System.out.println(response.toString());
require 'rest-client'
require 'json'
headers = {
'Accept' => 'application/json'
}
result = RestClient.get 'https://api.hippoassess.com/v1/json/{apiKey}/{emailAddress}/{ipAddress}/{firstName}/{lastName}',
params: {
}, headers: headers
p JSON.parse(result)
# © 2020, Email Hippo Limited. (http://emailhippo.com)
import requests
headers = {
'Accept': 'application/json'
}
r = requests.get('https://api.hippoassess.com/v1/json/{apiKey}/{emailAddress}/{ipAddress}/{firstName}/{lastName}', headers = headers)
print(r.json())
package main
import (
"bytes"
"net/http"
)
func main() {
headers := map[string][]string{
"Accept": []string{"application/json"},
}
data := bytes.NewBuffer([]byte{jsonReq})
req, err := http.NewRequest("GET", "https://api.hippoassess.com/v1/json/{apiKey}/{emailAddress}/{ipAddress}/{firstName}/{lastName}", data)
req.Header = headers
client := &http.Client{}
resp, err := client.Do(req)
// ...
}
/*
* © 2020, Email Hippo Limited. (http://emailhippo.com)
*/
using System;
using System.Collections.Generic;
using System.Net.Http;
using System.Net.Http.Headers;
using System.Text;
using System.Threading.Tasks;
using Newtonsoft.Json;
/// <<summary>>
/// Example of Http Client
/// <</summary>>
public class HttpExample
{
private HttpClient Client { get; set; }
/// <<summary>>
/// Setup http client
/// <</summary>>
public HttpExample()
{
Client = new HttpClient();
}
/// Make a dummy request
public async Task MakeGetRequest()
{
string url = "https://api.hippoassess.com/v1/json/{apiKey}/{emailAddress}/{ipAddress}/{firstName}/{lastName}";
var result = await GetAsync(url);
}
/// Performs a GET Request
public async Task GetAsync(string url)
{
//Start the request
HttpResponseMessage response = await Client.GetAsync(url);
//Validate result
response.EnsureSuccessStatusCode();
}
/// Deserialize object from request response
private async Task DeserializeObject(HttpResponseMessage response)
{
//Read body
string responseBody = await response.Content.ReadAsStringAsync();
//Deserialize Body to object
var result = JsonConvert.DeserializeObject(responseBody);
}
}
Performs email address verification to the full data enrichment level and returns the data in four formats:
JSON
GET //api.hippoassess.com/v1/json/{apiKey}/{emailAddress}/{ipAddress?}/{firstName?}/{lastName?}
BSON
GET //api.hippoassess.com/v1/bson/{apiKey}/{emailAddress}/{ipAddress?}/{firstName?}/{lastName?}
XML
GET //api.hippoassess.com/v1/xml/{apiKey}/{emailAddress}/{ipAddress?}/{firstName?}/{lastName?}
Protobuf
GET //api.hippoassess.com/v1/proto/{apiKey}/{emailAddress}/{ipAddress?}/{firstName?}/{lastName?}
GET ASSESS result.
Parameters
Parameter | In | Type | Required | Description |
---|---|---|---|---|
apiKey | path | string | true | the license key |
emailAddress | path | string | true | the email address |
ipAddress | path | string | false | the ip address |
firstName | path | string | false | the first name |
lastName | path | string | false | the last name |
Responses (including errors)
Status | Meaning | Description | Schema |
---|---|---|---|
200 | OK | Success | ResultRecord |
400 | Bad Request | Bad request. The server could not understand the request. Perhaps missing a license key or an email to check? Conditions that lead to this error are: No license key supplied, no email address supplied, email address > 255 characters, license key in incorrect format. | None |
401 | Unauthorised | Possible reasons: The provided license key is not valid, the provided license key has expired, you have reached your quota capacity for this account, this account has been disabled. | None |
429 | Too many requests | Maximum processing rate exceeded. See Concurrency for further information. | None |
500 | Internal Server Error | An error occurred on the server. Possible reasons are: license key validation failed or a general server fault. | None |
Result record schema
{
"version": {
"v": "ASSESS-(0.0.1)",
"doc": "https://docs.hippoassess.com/"
},
"meta": {
"lastModified": "string",
"expires": "string",
"email": "string",
"emailHashMd5": "string",
"emailHashSha1": "string",
"emailHashSha256": "string",
"firstName": "string",
"lastName": "string",
"domain": "string",
"ip": "string",
"tld": "string"
},
"hippoTrust": {
"score": 0
},
"userAnalysis": {
"syntaxVerification": {
"isSyntaxValid": true
},
"disposition": {
"isGibberish": true,
"isProfanity": true,
"gender": "string"
}
},
"emailAddressAnalysis":{
"mailboxVerification": {
"result": "string",
"secondaryResult": "string"
},
"syntaxVerification": {
"isSyntaxValid": true
},
"disposition": {
"isGibberish": true,
"isProfanity": true,
"isRole": true,
"isSubaddressing": true,
"isFreeMail": true,
"hasCatchAllServer": true,
"hasBeenBlocked": true
},
"assessments": {
"consumerBusiness": 0
}
},
"domainAnalysis":{
"disposition": {
"isSubDomain": true,
"isGibberish": true,
"isProfanity": true,
"isDarkWeb": true,
"hasBeenBlocked": true,
"hasDnsRecords": true,
"hasMxRecords": true,
"mailServerLocation": "string",
"mailServerInfrastructure": "string"
},
"age": {
"text": "string",
"seconds": 0,
"iso8601": "string"
},
"registration": {
"ownerContactName": "string",
"ownerContactCity": "string",
"ownerContactCountry": "string"
}
},
"ipAddressAnalysis":{
"disposition": {
"isTorExitNode": true,
"hasBeenBlocked": true
},
"location": {
"latitude": 0,
"longitude": 0,
"timeZone": "string",
"continent":{
"code":"string",
"name":"string"
},
"country":{
"code":"string",
"name":"string",
"isInEU": true,
"currencies": [
"string"
],
"dialingCodes": [
"string"
]
},
"city":{
"name":"string"
}
},
"dataCenter":{
"autonomousSystemNumber": 0,
"autonomousSystemOrganization": "string",
"connectionType": "string",
"isp": "string",
"organization": "string"
}
}
}
Name | Type | Required | Restrictions | Description | Example use case |
---|---|---|---|---|---|
Version | |||||
v | string | false | none | Contains details of the version and edition of API | |
doc | string | false | none | ||
Meta | |||||
lastModified | string | false | none | Last modified date/time of Email Hippo record | |
expires | string | false | none | Date/time that this record expires from Email Hippo cache | |
string | false | none | The email being queried | ||
emailHashMd5 | string | false | none | MD5 hash of the email address | |
emailHashSha1 | string | false | none | SHA1 hash of the email address | |
emailHashSha256 | string | false | none | SHA265 hash of the email address | |
firstName | string | false | none | The first name submitted | |
lastName | string | false | none | The last name submitted | |
domain | string | false | none | The domain of the email being queried | |
ip | string | false | none | The ip address submitted | |
tld | string | false | none | The Top Level Domain (TLD) of email being queried | |
Hippo Trust | |||||
score | decimal | false | none | How much can I trust the person associated with this email address and ip | |
User Analysis | |||||
disposition | |||||
isGibberish | boolean | false | none | Is the first name or last name deemed to be gibberish text? | |
isProfanity | boolean | false | none | Is the first name or last name deemed to be profanity? | |
gender | string | false | none | The gender been identified from the first name or email address | |
Email Address Analysis | |||||
mailboxVerification | |||||
result | string | false | none | Primary result codes | |
secondaryResult | string | false | none | Secondary result codes | |
syntaxVerification | |||||
isSyntaxValid | boolean | false | none | Is the syntax of the email address correct according to RFC standards? | |
disposition | |||||
isGibberish | boolean | false | none | Is the email address deemed to be gibberish text? | |
isProfanity | boolean | false | none | Is the email address deemed to be profanity? | |
isRole | boolean | false | none | Is a role address? (e.g. info@, sales@, postmaster@) | |
isSubaddressing | boolean | false | none | Does the email contain subaddressing i.e. + some text or (some text) | |
isFreemail | boolean | false | none | Is a free mail provider? (e.g. gmail, hotmail etc) | |
hasCatchAllServer | boolean | false | none | Is the mail server a catch all mail server? | |
hasBeenBlocked | boolean | false | none | Is the email address on a reputable block list? | |
assessments | |||||
consumerBusiness | decimal | false | none | Does the email address relate to a consumer or a business? Consumer 0-4, Business 6-10 | |
Domain Analysis | |||||
disposition | |||||
isSubDomain | boolean | false | none | Is a subdomain being used in the email address? | |
isGibberish | boolean | false | none | Is the domain deemed to be gibberish text? | |
isProfanity | boolean | false | none | Is the domain deemed to be profanity? | |
isDarkWeb | boolean | false | none | Has the domain been associated with the Dark Web? | |
hasBeenBlocked | boolean | false | none | Is the domain on a reputable block list? | |
hasDnsRecord | boolean | false | none | Does the domain have any DNS records? | |
hasMxRecords | boolean | false | none | Does the domain have any MX records? | |
mailServerLocation | string | false | none | Mail server location as a 2 digit ISO code (e.g. US) | |
mailServerInfrastructure | string | false | none | Infrastructure Identifier (e.g. Hotmail, GoogleforBiz, Office365, Mimecast) | |
age | |||||
text | string | false | none | Age of the domain in human readable text | |
seconds | integer | false | none | Age of the domain in seconds | |
iso8601 | string | false | none | Age of the domain in iso8601 format | |
registration | |||||
ownerContactName | string | false | none | The domain owner's contact name | |
ownerContactCity | string | false | none | The domain owner's contact city | |
ownerContactCountry | string | false | none | The domain owner's contact country | |
IP Address Analysis | |||||
disposition | |||||
isTorExitNode | boolean | false | none | Is the ip address a Tor exit node? | |
hasBeenBlocked | boolean | false | none | Is the ip address on a reputable block list? | |
location | |||||
latitude | decimal | false | none | The latitude of the ip address's location | |
longitude | decimal | false | none | The longitude of the ip address's location | |
timeZone | string | false | none | The timeZone of the ip address's location | |
continent | |||||
code | string | false | none | The 2 char code for the continent of the ip address's location | |
name | string | false | none | The English name for the continent of the ip address's location | |
country | |||||
code | string | false | none | The 2 char ISO code for the country of the ip address's location | |
name | string | false | none | The English name for the country of the ip address's location | |
isInEU | boolean | false | none | Is the country in the European Union? | |
currencies | string[] | false | none | The currency codes for the country | |
callingCodes | string[] | false | none | The international dialing/calling codes for the country/territories | |
city | |||||
name | string | false | none | The English name for the city of the ip address's location | |
dataCenter | |||||
autonomousSystemNumber | integer | false | none | The ASN for the data center | |
autonomousSystemOrganization | string | false | none | The ASN's organisation name | |
connectionType | string | false | none | The connection type (e.g. Corporate, Residential) | |
isp | string | false | none | The name of the isp | |
organization | string | false | none | The isp's Organisation name |
Result codes
Primary mailbox result codes
Primary code | Description |
---|---|
Ok | Verification passes all checks including Syntax, DNS, MX, Mailbox, Deep server configuration, Greylisting. |
Bad | Verification fails checks for definitive reasons (e.g. mailbox does not exist). |
Disposable email address | The email address is provided by a well known disposable provider |
Unverifiable | Conclusive verification result cannot be achieved due to mail server configuration or anti-spam measures. |
Secondary mailbox result codes
Primary code | Secondary reason | Description |
---|---|---|
Ok | Success | Successful verification. 100% confidence that the mailbox exists. |
Bad | DomainDoesNotExist | The domain (i.e. the bit after the ‘@’ character) defined in the email address does not exist, according to DNS records. A domain that does not exist cannot have email boxes. |
Bad | EmailAddressFoundOnBlockLists | The email address is found on one or more block lists. |
Bad | EmailContainsInternationalCharacters | Email address contains international characters. |
Bad | InvalidEmailFormat | Invalid email address format. |
Bad | MailboxDoesNotExist | The mailbox does not exist. 100% confidence that the mail box does not exist. |
Bad | MailboxFull | Mailboxes that are full are unable to receive any further email messages until such time as the user empties the mail box or the system administrator grants extra storage quota. Most full mailboxes usually indicate accounts that have been abandoned by users and will therefore never be looked at again. We do not recommend sending emails to email addresses identified as full. |
Bad | MailServerFaultDetected | An unspecified mail server fault was detected. |
Bad | NoMailServersForDomain | There are no mail servers defined for this domain, according to DNS. Email addresses cannot be valid if there are no email servers defined in DNS for the domain. |
Bad | PossibleSpamTrapDetected | A possible spam trap email address or domain has been detected. Spam traps are email addresses or domains deliberately placed on-line in order to capture and flag potential spam based operations. Our advanced detection heuristics are capable of detecting likely spam trap addresses or domains known to be associated with spam trap techniques. We do not recommend sending emails to addresses identified as associated with known spam trap behaviour. Sending emails to known spam traps or domains will result in your ESP being subjected to email blocks from a DNS Block List. An ESP cannot tolerate entries in a Block List (as it adversely affects email deliverability for all customers) and will actively refuse to send emails on behalf of customers with a history of generating entries in a Block List. |
Disposable email address | DomainIsWellKnownDea | The domain is a well known Disposable Email Address DEA. There are many services available that permit users to use a one-time only email address. Typically, these email addresses are used by individuals wishing to gain access to content or services requiring registration of email addresses but same individuals not wishing to divulge their true identities (e.g. permanent email addresses). DEA addresses should not be regarded as valid for email send purposes as it is unlikely that messages sent to DEA addresses will ever be read. |
Unverifiable | MailServerAntiSpam | The email server is using anti-spam measures. Anti-spam systems and processes can include: proxy based, hosted anti-spam such as ProofPoint or MimeCast; self hosted anti-spam using remote data services such as Barracuda, SORBS or SPAMCOP; and Whitelist based systems only allow emails from pre-approved sender email address. Pre-approval of sender email addresses can be done manually by the email system administrator and/or automatically via "click this link" to confirm your email address type opt-in emails. |
Unverifiable | MailServerGreyListing | Greylisting is in operation. It is not possible to validate email boxes in real-time where greylisting is in operation. |
Unverifiable | MailServerCatchAll | The server is configured for catch all and responds to all email verifications with a status of Ok. Mail servers can be configured with a policy known as Catch All. Catch all redirects any email address sent to a particular domain to a central email box for manual inspection. Catch all configured servers cannot respond to requests for email address verification. |
Unverifiable | None | No additional information is available. |
Unverifiable | Timeout | The remote email server timed out. Mail server is too busy / overloaded, misconfigured, offline or otherwise unavailable. |
Unverifiable | UnpredictableSystem | Unpredictable system infrastructure detected. Various email services deliver unpredictable results to email address verification. The reason for this unpredictability is that some email systems elect not to implement email standards (i.e. RFC 2821). For systems that are known to be unpredictable, we return a secondary status of 'UpredictableSystem'. |
Email Hippo Trust Score
The Trust Score provides an ‘at a glance’ determination of quality; drilling deeper than just the email address itself.
The Email Hippo Trust Score is designed to answer a fundamental question posed from the perspective of a business owner, merchant, data broker or lead generation service:
- How much can I trust the person associated with this email address and optionally ip address?
The Trust Score takes dozens of metrics and signals into consideration when making this assessment and providing the final score.
Consumer Business assessment
The Consumer Business Assessment rates the liklihood that the email address belongs to a consumer or a business.
The closer the score is to 0 the more likely the email address relates to a consumer.
The closer the score is to 10 the more likely the email address relates to a business.
.Net client libraries
We have a .NET package built for easy integration with our ASSESS Edition 1 (v1) API services. For further information on the RESTful server side implementation, please see the docs and schema.
If you're working in the .NET environment, this package can save you hours of work writing your own JSON parsers, message pumping logic, threading and logging code.
How to get the package
The package is available from Nuget.
Frequently asked questions
How can I get a key?
Click here to sign up and generate a key.
Can I trust you with my data?
Great question. Yes, of course - see our Compliance pages for detailed information.
Will I get blocklisted using your APIs?
No. It’s Email Hippo infrastructure that does the work.
Will anyone know that I am querying an email address, a domain or an ip address?
No. It’s Email Hippo infrastructure that does the work.
How do I call your APIs?
For a JSON response, make a simple GET request to the endpoint.
Read the endpoint sections for more information and take a look at the code samples, or try it out when you sign up.
Does the system get slower when it’s busy?
No. All infrastructure is hosted in cloud based platforms with automatic scaling enabled. Automatic scaling kicks in at busy times to provide more hardware resources to meet demand.
Do you cache results?
To deliver the speed and reliability demanded by our customers, verification results are cached as follows:
Level 1 cache: CloudFlare based. Cache expiration 2 hours.
Level 2 cache: Microsoft Azure based - see table below
Service | Cache expiration |
---|---|
ASSESS Edition 1/V1 | Up to 30 days |
No personally identifiable information is stored in our cache infrastructure.
Can I get my usage in real-time?
Usage is available in the Hippo World customer portal or through the ASSESS quota API.
What comes back from the API?
Various text or binary response formats.
Note: For a detailed explanation of the responses available, see the appropriate service Edition/Version Schema on this site.
Glossary
ACL
Access Control List.
An ACL determines what networking traffic is allowed to pass and what traffic is blocked.
An ACL change is sometimes required to your company firewall in order to access our API.
API
Application Programmers Interface.
See Wikipedia - API Definition for further information.
B2B
Business To(2) Business
Business email hosting services are generally private, enterprise grade hosting services typically hosted in either private data centers or in cloud based infrastructure.
Business to business refers to the activity of businesses sending email to clients using business email addresses.
B2C
Business To(2) Consumer
Consumer email hosting providers are generally well known, mostly web based providers such as Outlook, Yahoo, AOL, Gmail etc.
Business to consumer refers to the activity of businesses sending email to clients using consumer email addresses.
Verifying email addresses in consumer domains is generally more technically challenging than B2B
Block list
See DNSBL.
BSON
Binary Object Notation
See Wikipedia - BSON for further information.
CORS
Cross Origin Resource Scripting
Allows modern browsers to work with script (e.g. JavaScript) and JSON data originating form other domains.
CORS is required to allow client script such a JavaScript, jQuery or AngularJS to work with results returned from an external RESTful API.
See Wikipedia - CORS for more information.
DDoS
Distributed Denial of Service
See Wikipedia - Denial-of-service attack for further information.
DEA
Disposable Email Address
There are many services available that permit users to use a one-time only email address. Typically, these email addresses are used by individuals wishing to gain access to content or services requiring registration of email addresses but same individuals not wishing to divulge their true identities (e.g. permanent email addresses).
DEAs should not be regarded as valid for email send purposes as it is unlikely that messages sent to DEAs will ever be read.
DNS
Domain Name System
At its simplest level, DNS converts text based queries (e.g. a domain name) into IP addresses.
DNS is also responsible for providing the MX records needed to locate a domains mail servers.
See Wikipedia - Domain Name System for further information.
DNSBL
DNS Block List
As an anti-spam measure, mail servers can use spam block lists to ‘look up’ the reputation of IP addresses and domains sending email. If an IP or domain is on a block list, the mail server may reject the senders email message.
See Wikipedia - DNSBL for further information.
ESP
Email Service Provider
A service that sends emails on your behalf.
See Wikipedia - Email service provider (marketing) for more information.
Free mail
Addresses served by popular B2C service providers such as Outlook, Yahoo, Live, AOL, Gmail and so on.
Greylisting
A technique used in mail servers as an anti-spam technique. Sometimes also known as “deferred”, greylisting arbitrarily delays the delivery of emails with a “try again later” response to the client sending the email.
See Wikipedia - Greylisting for more information.
HTTP
Hypertext Transfer Protocol
See Wikipedia - Hypertext Transfer Protocol for more information.
IP address
Internet Protocol Address
See Wikipedia - IP Address for more information.
ISO 3166
International standard for country codes.
See Country Codes - ISO 3166 for more information.
JSON
JavaScript Object Notation
JavaScript Object Notation, is an open standard format that uses human readable text to transmit data objects consisting of attribute value pairs. It is used primarily to transmit data between a server and web application.
See Wikipedia - JSON for more information.
License key
License key authentication is best for situations where simplicity is required and you can keep the key private. An ideal use case for key authentication would be for server based applications calling the RESTful API.
Click here to sign up and access a license key.
ms
Milliseconds.
MX
Mail Exchanger
The MX is a server responsible for email interchange with a client.
NDR
Non Delivery Report
A message that is returned to sender stating that delivery of an email address was not possible.
See Wikipedia - Bounce message for more information.
Office 365
Office 365 mail servers (e.g. x-com.mail.protection.outlook.com) are always configured with the catch all policy, accepting all emails sent to the domain and redirecting them to a central email box for manual inspection. Catch all configured servers cannot respond to requests for email address verification.
This does not affect our coverage of Hotmail, Live and Outlook mailboxes.
protobuf
Protocol Buffers is a method of serializing structured data.
See Wikipedia - Protocol Buffers for more information.
Punycode
Punycode is a way to represent Unicode with the limited character subset of ASCII supported by the Domain Name System.
See Wikipedia - Punycode for more information.
RESTful
Representational state transfer
See Wikipedia - RESTful for more information.
RFC
Request for Comments
The principal technical development and standards-setting bodies for The Internet.
See Wikipedia - Request for Comments for more information.
Role address
A role address is a generic mailbox such as info@
Role addresses allow collaborative working based on groups rather than indiviidual mailboxes.
SLA
Service Level Agreement
See Wikipedia - SLA for more information.
See our Service Level Agreement.
SMTP
Simple Mail Transport Protocol
SMTP is a protocol. It is the sequence of commands and responses between a client (the software sending an email) and server (the software receiving an email) that facilitates the sending and receiving of email between computer based email messaging systems.
Spam trap
Spam traps are email addresses used for the sole purpose of detecting spamming activities.
Spam traps are used by many block lists (DNSBL) to detect spammers.
See Wikipedia - Spam traps for more information.
TXT
TXT records associate arbitary and unformatted text with a domain. TXT records uses include Sender Policy Framework (SPF) and other domain validation applications.
See Wikipedia - TXT record for more information.
XML
e(X)tensible Markup Language
See Wikipedia - XML for more information.